Ensuring the regular update of Docker containers is crucial for sustaining safe, efficient, and dependable software environments. To avoid downtime and mitigate possible security threats, it is crucial to have a comprehensive grasp of how to efficiently update Docker containers. This includes renewing a base image, altering application code, or upgrading the whole Docker system. This tutorial will explore the many techniques and recommended approaches for upgrading Docker containers. It will include practical examples and valuable insights to ensure that you are well-prepared to manage these tasks.
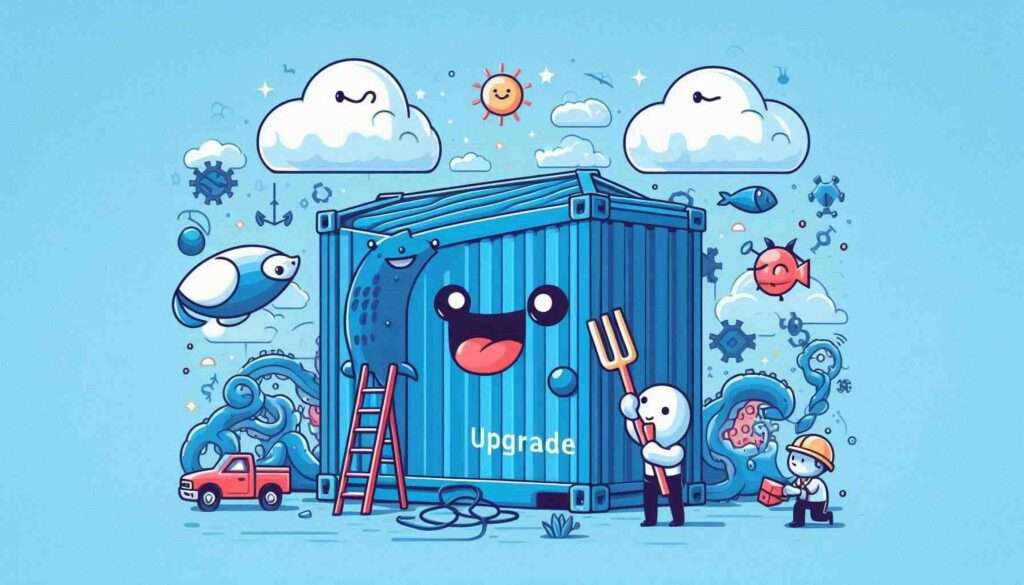
Why Updating Docker Containers Matters
Docker containers have transformed the process of deploying and overseeing applications, offering uniform environments across the stages of development, testing, and production. Nevertheless, similar to any other software environment, Docker containers have the potential to become obsolete. This might be attributed to the introduction of recent iterations of the foundational image, modifications made to the application code, or the implementation of patches aimed at resolving security issues.
By updating your Docker containers, you can guarantee that your applications are operating on the most up-to-date software. This may result in better speed, the addition of new features, and, most critically, improved security. Failing to update your apps might leave them vulnerable to security breaches and compatibility problems.
Understanding Docker Image Updates
A Docker image is what your container is built on. Along with the code, runtime, libraries, and environment factors, it has everything an application needs to run. A common task is to update a Docker image, especially when new versions of the base image or your application code come out.
A Docker image is usually updated by downloading the most recent version, checking it for any changes or possible issues, and then deploying it in place of the old image. This method makes sure that the components in your containers are always running the most up-to-date and safe versions.
Pulling the Latest Image
To begin, you need to get the most recent version of the file from a site like Docker Hub. The docker pull
command gets the most recent version of the image and saves it on your computer. If you’re working on a Node.js application, for example, you could get the most recent Node.js picture by using:
docker pull node:14
Inspecting the Image
It is important to check the new picture carefully before deploying it. You can find any changes that might affect your application in this step. To check out the environment, you can run a test container from the new image:
docker run -it --rm node:14 bash
You can check that the environment is set up properly by using this command to open a live prompt inside the container.
Updating the Dockerfile and Rebuilding the Image
If your application sets up the container environment with a Dockerfile, you’ll need to change the FROM
command to point to the new image version. Now that you’ve made this change, rebuild your Docker image:
docker build -t mynodeapp:latest .
It is very important to test the new picture before putting it into production. Make sure everything works right after running the new container. Once you’re satisfied, you can replace the old container with the new one, using commands like:
docker stop mynodeapp
docker rm mynodeapp
docker run -d --name mynodeapp -p 80:80 mynodeapp:latest
This approach minimizes disruptions by ensuring the updated container is thoroughly tested before being deployed.
Updating Code Within a Docker Container
Sometime, getting a new image isn’t enough to update a container; you also need to change the application code that’s running inside the container. To do this, you can either rebuild the container with the new code or use Docker volumes for a more dynamic, real-time update process.
Rebuilding the Container with Updated Code
A safe way is to rebuild the container with new code. This is especially true in production settings where stability is very important. The first step is to update your source locally. If you make changes to your code that require new variables or changes to the environment, you should make those changes to your Dockerfile. After that, rebuild the Docker image and run the container that has been changed. This method makes sure that all dependencies are installed properly and that the environment stays the same from one release to the next.
If you’re changing a Python application, for instance, you might first update the code and then change the Dockerfile to match any new dependencies:
FROM python:3.9-slim
WORKDIR /app
COPY . /app
RUN pip install -r requirements.txt
CMD ["python", "app.py"]
Once the Dockerfile is updated, rebuild the image and deploy the new container:
docker build -t mypythonapp:v2 .
docker stop mypythonapp
docker rm mypythonapp
docker run -d --name mypythonapp -p 5000:5000 mypythonapp:v2
Testing the updated container is crucial to ensure that the new code functions correctly and that there are no issues in the updated environment.
Using Docker Volumes for Real-Time Code Updates
It’s more flexible to use Docker volumes to put your local code path into the container in development settings where you may want to see the effects of changes to the code right away. This lets you change the code right away, without having to restart the container each time.
To do this, start the Docker container with the -v
flag to mount the local code directory:
docker run -d -v /path/to/local/code:/app -p 5000:5000 mypythonapp
As you make changes to your code locally, these changes are reflected in the running container. Depending on your application, you might need to restart the container to apply the changes. For example:
docker restart mypythonapp
This approach speeds up development by allowing immediate feedback on code changes, making it easier to iterate and refine your application.
Best Practices for Updating Docker Containers in Production
To avoid downtime and make sure the move goes smoothly, updating Docker containers in a production environment needs to be carefully planned and carried out. Blue-green deployments and rolling changes are common ways to keep services available and cause as little trouble as possible.
Blue-Green Deployment Strategy
The blue-green distribution method keeps two similar settings, called blue and green. The latest version is running in one environment, and the improved version is ready to go in the other. By using this method, you can quickly switch between settings.
To set up a blue-green release, you must first send the new container along with the current one. You can move traffic from the old environment to the new one after a lot of testing. This could mean changing the settings for a load broker or updating DNS records. The old environment can be shut down once the new one is safe.
If issues arise with the new release, this approach gives you a safety net that lets you go back to the old environment.
Rolling Updates with Orchestration Tools
When using container orchestration systems such as Docker Swarm or Kubernetes, rolling updates provide an automated method to update containers while minimizing the effect on service availability.
The docker service update
command in Docker Swarm allows for the progressive replacement of old containers with new ones when updating a service. This guarantees the continuous availability of the service throughout the upgrade process.
The kubectl set image
command is used in Kubernetes to modify containers inside a deployment. Kubernetes will manage the deployment process by systematically replacing outdated pods with updated ones.
Both of these technologies are well-suited for large-scale contexts where ensuring continuous operation is of utmost importance.
Managing Dependencies During Docker Container Updates
When performing updates on Docker containers, it is essential to manage dependencies, especially when working with intricate applications. Ensuring that all dependencies are up-to-date and compatible with your application is essential for the success of the upgrade.
Auditing Dependencies
Check your current sources to see if any of them need to be updated before you update your container. Npm audit
, for instance, can be used to find security holes and out-of-date tools in a Node.js application. When you use Python, pip list --outdated
can help you find tools that need to be updated.
Updating Dependencies in the Dockerfile
You must change your Dockerfile if your application depends on external libraries or packages. To do this, you might need to change the RUN
scripts that install dependencies or bring the package manager up to date.
For example, if you’re using a Python application, you might change the requirements.txt
file and then the Dockerfile to load the new packages:
RUN pip install --upgrade -r requirements.txt
Once you’re done with these changes, restart your Docker image and run the new container. To make sure that the changed requirements don’t bring any new issues or conflicts, testing is important.
Real-World Example: Updating a Dockerized Web Application
Consider a real-life situation where you need to update a Dockerized web application. This will show you how to update a Docker container. You just got a security patch that needs to be pushed to the Node.js server where this application runs.
The first thing you need to do is get the most recent Node.js version that has the security patch:
docker pull node:14
Next, update your Dockerfile to use the latest image:
FROM node:14
WORKDIR /app
COPY . /app
RUN npm install
CMD ["node", "server.js"]
Rebuild your Docker image with the updated Dockerfile:
docker build -t mywebapp:latest .
Before deploying the updated container, run it in a test environment to ensure that everything works as expected:
docker run -d --name mywebapp-test -p 8080:80 mywebapp:latest
After thorough testing, you can stop the old container and replace it with the new one:
docker stop mywebapp
docker rm mywebapp
docker run -d --name mywebapp -p 80:80 mywebapp:latest
This process ensures that your web application is running the latest, most secure version without disrupting service.
Conclusion: Mastering Docker Container Updates
Maintaining the latest versions of Docker containers is an essential practice for ensuring the security, efficiency, and reliability of applications. By comprehending the diverse techniques and optimal strategies for upgrading Docker containers, you can guarantee that your software environment stays at the forefront and safeguarded from vulnerabilities.
Every step in the update process, such as fetching the newest image, changing application code, or managing dependencies, is essential for ensuring the overall well-being of your Dockerized apps. By adhering to the instructions and illustrations shown in this article, you may securely and effectively upgrade your Docker containers, guaranteeing seamless and continuous service for your users.
Frequently Asked Questions(FAQ) about Docker Containers
Q: What happens if I update a Docker container while it’s running?
A: Usually, to update a running container, you have to stop it, delete it, and then start a new one with the updated picture. You can’t directly change the picture of a live container without starting over with the container.
Q: Can I update the code in a Docker container without rebuilding the image?
A: Yes, you can use Docker volumes to mount your local code directory into the container, allowing real-time updates. This method is useful for development environments but is not recommended for production.
Q: How do I know when a Docker image needs to be updated?
A: Regularly check the image source, such as Docker Hub, for updates. You can also subscribe to notifications from the image maintainers. Additionally, tools like docker inspect
and docker image ls
can help you track image versions.
Q: What are the risks of not updating Docker containers?
A: Failure to update Docker containers might expose your application to security vulnerabilities, impair performance owing to obsolete dependencies, and result in compatibility conflicts with other software components.
Q: How can I automate Docker container updates?
A: Utilize orchestration solutions such as Docker Swarm or Kubernetes, which provide automatic updating procedures like rolling updates. In addition, CI/CD pipelines may be set up to automatically reconstruct and redeploy containers as changes become accessible.